Enable Caching In Core PHP
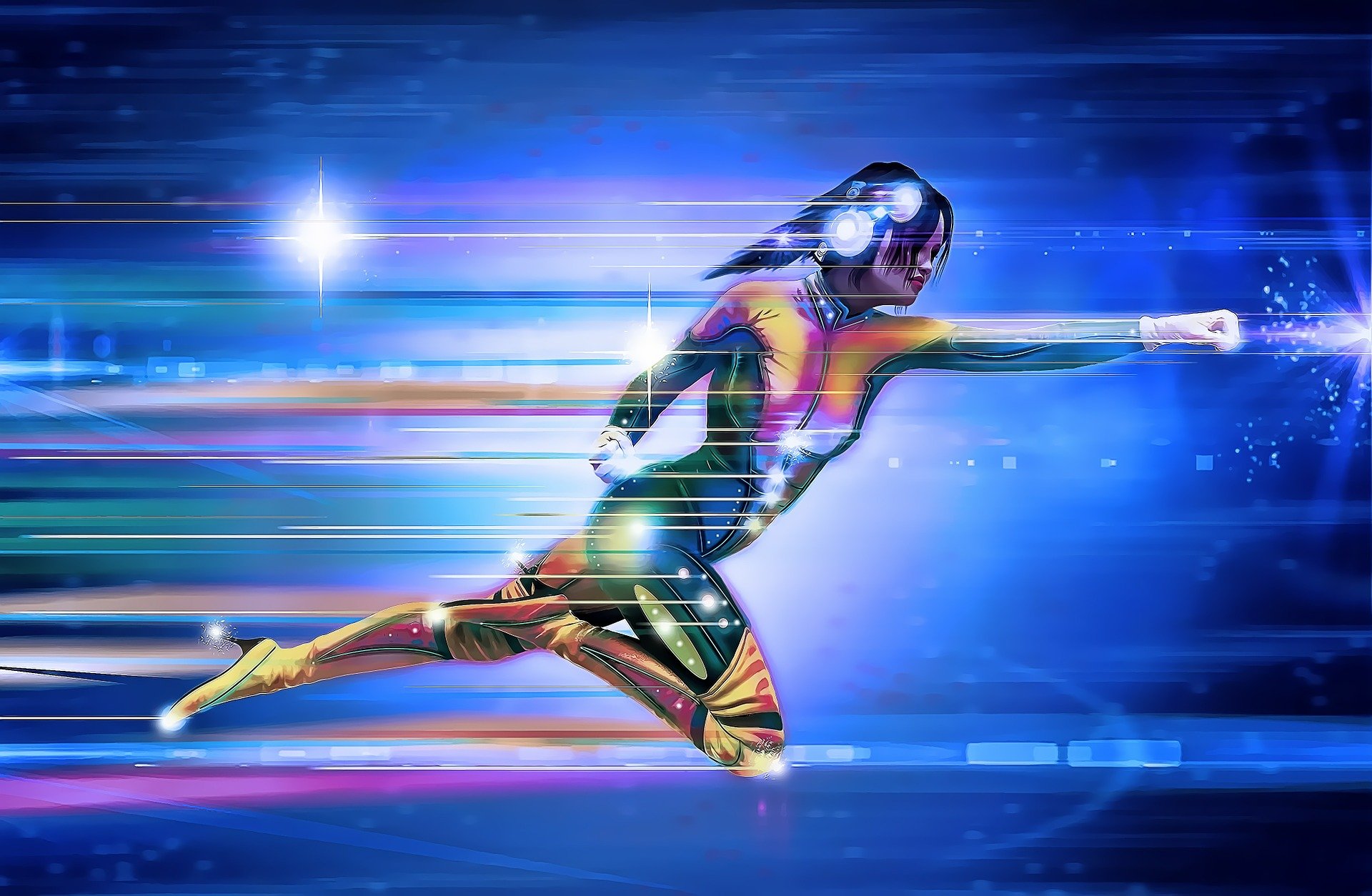
Caching is a recommended feature to improve website loading speed. CMS like WordPress provides caching with plugins. But in Core PHP and frameworks (most of them) it’s hard to implement.
Before going to learn about the methods, we first dig about cache and why it’s an important factor.
Table of Contents
What is Caching?
When a URL is hit, it sends a request to the server and renders the response. Meanwhile, it creates many requests to the server. Like it fetches data from the database. The server renders data with help of a server language (like PHP). It makes multiple requests every time a URL is hit.
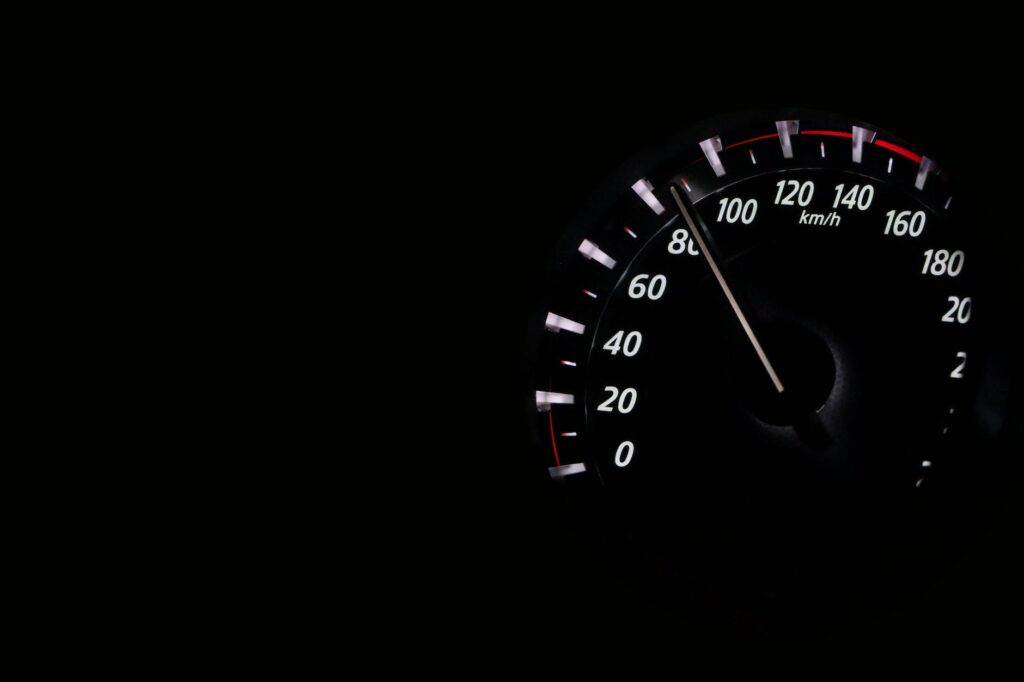
Caching stores the final result (cached) of the rendered document somewhere on the server. On next time, the URL is hit, it generates a webpage via the cached file. So, multiple requests are bye-passed and ultimately page loads faster.
Caching is progressive
As caching bye-pass the original content and renders the cached page, sometimes it creates a problem.
If some content is updated on the page, but due to caching, it shows an older version. So it’s necessary to update the cached version on a timely basis.
Enable caching in the Core PHP website
There are two methods to enable caching on the core PHP website.
Cache a website with a .htaccess file
Caching with htaccess enables Browser Caching. Browser caching means assets added to a webpage are stored in the browser’s memory. So next time, the reader visit the same webpage again, the browser doesn’t need to download the media, CSS and Scripts file again.
.htaccess file generally exits on the root folder. If you don’t find it, just create it. If it has content, then just add the below code to the last of the file.
# BEGIN Expire headers
<IfModule mod_expires.c>
# Turn on the module.
ExpiresActive on
# Set the default expiry times.
ExpiresDefault "access plus 2 days"
ExpiresByType image/jpg "access plus 1 month"
ExpiresByType image/svg+xml "access 1 month"
ExpiresByType image/gif "access plus 1 month"
ExpiresByType image/jpeg "access plus 1 month"
ExpiresByType image/png "access plus 1 month"
ExpiresByType text/css "access plus 1 month"
ExpiresByType text/javascript "access plus 1 month"
ExpiresByType application/javascript "access plus 1 month"
ExpiresByType application/x-shockwave-flash "access plus 1 month"
ExpiresByType image/ico "access plus 1 month"
ExpiresByType image/x-icon "access plus 1 month"
ExpiresByType text/html "access plus 600 seconds"
</IfModule>
# END Expire headers
Caching with PHP
In the method, some HTML files are generated on server and rendered instead of the original one.
These files have a lifeline. After that period, cached files are updated with the latest content. So readers will get the latest content.
To implement PHP caching, follow below steps.
Create top-cache.php File
Create top-cache.php file on the root folder and add the following code.
<?php
$url = $_SERVER["SCRIPT_NAME"];
$break = Explode('/', $url);
$file = $break[count($break) - 1];
$cachefile = 'cached/cached-'.substr_replace($file ,"",-4).'.html';
$cachetime = 18000;
// Serve from the cache if it is younger than $cachetime
if (file_exists($cachefile) && time() - $cachetime < filemtime($cachefile)) {
echo "<!-- Cached copy, generated ".date('H:i', filemtime($cachefile))." -->\n";
readfile($cachefile);
exit;
}
ob_start(); // Start the output buffer
?>
Create bottom-cache.php File
Create bottom-cache.php file on root folder and add below code.
<?php
// Cache the contents to a cache file
$cached = fopen($cachefile, 'w');
fwrite($cached, ob_get_contents());
fclose($cached);
ob_end_flush(); // Send the output to the browser
?>
Include Files On Your Page
Now you have to include these files to implement caching.
<?php
include('top-cache.php');
// Your regular PHP code goes here
include('bottom-cache.php');
?>
All set.
On every unique page visited, HTML file is generated in the cached folder. Which render first and will re-generate every 1800 seconds.